Almost every web site or web application I know have a common layout shared among all the pages. To avoid copy and paste programming, developers usually look for a web template system. Tiles is one of them, made to be used in a J2EE context. It is an implementation of the View Composite core J2EE pattern. I’ll show a brief example to get started with Tiles 2.1.
Typically, Tiles will be installed and configured in a J2EE web application. To begin, I will only create a simple, typical layout. But let’s configure the framework first.
Configuration
There are many ways of configuring Tiles. In my example, I’ll do it like this :
<?xml version="1.0" encoding="ISO-8859-1"?> <web-app xmlns="http://java.sun.com/xml/ns/javaee" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" version="2.5"> <context-param> <param-name> org.apache.tiles.definition.DefinitionsFactory.DEFINITIONS_CONFIG </param-name> <param-value> /WEB-INF/myTilesConfigFile.xml </param-value> </context-param> <listener> <listener-class>org.apache.tiles.web.startup.TilesListener</listener-class> </listener> <welcome-file-list> <welcome-file>home.jsp</welcome-file> </welcome-file-list> </web-app>
This way, I’m telling my application that I’m using Tiles. It is the configured Context listener that will bootstrap Tiles. The specified context parameter tells the listener in which XML file pages definitions will be.
This XML file defines all existing pages of my application. I specified above that this file will be named myTilesConfigFile.xml, and will be placed under WEB-INF. Here is the content of the definition file :
<?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE tiles-definitions PUBLIC "-//Apache Software Foundation//DTD Tiles Configuration 2.0//EN" "http://tiles.apache.org/dtds/tiles-config_2_0.dtd"> <tiles-definitions> <definition name="homePage" template="/template.jsp"> <put-attribute name="header" value="/defaultHeader.jsp" /> <put-attribute name="menu" value="/defaultMenu.jsp" /> <put-attribute name="body" value="/home_body.jsp" /> <put-attribute name="footer" value="/defaultFooter.jsp" /> </definition> </tiles-definitions>
As the code says, I define a page and all its parts, specifying a name for the definition itself, and a template to use.
The template
I want most of my pages to share the same header, footer and left menu. The template file is called template.jsp and is placed under the web application’s root directory :
template.jsp
<%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles" %> <html> <body style="width:100%;height:100%"> <table border="1" cellspacing="0" cellpadding="0" style="width:100%;height:100%"> <tr> <td colspan="2"> <tiles:insertAttribute name="header" /></td> </tr> <tr> <td> <tiles:insertAttribute name="menu" /></td> <td> <tiles:insertAttribute name="body" /></td> </tr> <tr> <td colspan="2"> <tiles:insertAttribute name="footer" /></td> </tr> </table> </body> </html>
Layout parts
header (defaultHeader.jsp)
<div>this is the default header</div>
footer (defaultFooter.jsp)
<div>This is the default footer...</div>
menu (defaultMenu.jsp)
<div> <ul> <li>Menu item 1</li> <li>Menu item 2</li> <li>Menu item 3</li> <li>Menu item 4</li> <li>Menu item 5</li> <li>Menu item 6</li> </ul> </div>
body (home_body.jsp)
<div id="header"> <h1>This is the home page's body</h1> </div>
Now I have a template and all the pieces needed to fill it. But I don’t have the home page itself.
home page (home.jsp)
Here is the code for the home page named “home.jsp” :
<%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles" %> <tiles:insertDefinition name="homePage" />
This page only tells Tiles which definition to use. Then Tiles can read that definition to find which template to take, and for each part of the template, what to insert. To avoid confusion, here is how the directory tree now looks like :
tiles |-- WEB-INF | |-- lib | | |-- commons-beanutils-1.8.0.jar | | |-- commons-digester-1.8.1.jar | | |-- commons-logging-api-1.1.jar | | |-- tiles-api-2.1.2.jar | | |-- tiles-core-2.1.2.jar | | |-- tiles-jsp-2.1.2.jar | | `-- tiles-servlet-2.1.2.jar | |-- myTilesConfigFile.xml | `-- web.xml |-- defaultFooter.jsp |-- defaultHeader.jsp |-- defaultMenu.jsp |-- home.jsp |-- home_body.jsp `-- template.jsp
Starting the application server and accessing the home.jsp will produce the expected output :
To make it clearer, let’s now define a second page that will use the same template. What I’m thinking of is an about page. First, write the definition in the myTilesConfigFile.xml configuration file :
<?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE tiles-definitions PUBLIC "-//Apache Software Foundation//DTD Tiles Configuration 2.0//EN" "http://tiles.apache.org/dtds/tiles-config_2_0.dtd"> <tiles-definitions> <definition name="homePage" template="/template.jsp"> <put-attribute name="header" value="/defaultHeader.jsp" /> <put-attribute name="menu" value="/defaultMenu.jsp" /> <put-attribute name="body" value="/home_body.jsp" /> <put-attribute name="footer" value="/defaultFooter.jsp" /> </definition> <definition name="aboutPage" template="/template.jsp"> <put-attribute name="header" value="/defaultHeader.jsp" /> <put-attribute name="menu" value="/defaultMenu.jsp" /> <put-attribute name="body" value="/about_body.jsp" /> <put-attribute name="footer" value="/defaultFooter.jsp" /> </definition> </tiles-definitions>
Now there is much less missing parts; I only have to code the page’s body, and the page itself.
about_body.jsp
<h1>About us</h1> Lorem ipsum dolor sit amet, consectetur adipiscing elit. Morbi tempus mauris condimentum arcu. Nunc in quam. Vivamus quis quam sed tortor euismod pellentesque. Nulla lobortis. Donec urna metus, adipiscing vel, iaculis vel, congue at, odio. Nullam et dolor id tortor tempor gravida. Curabitur eleifend
about.jsp
<%@ taglib uri="http://tiles.apache.org/tags-tiles" prefix="tiles" %> <tiles:insertDefinition name="aboutPage" />
And finally, to make all this example a little bit more consistent, I edit my menu to put links on the home and about pages :
<div> <ul> <li><a href="/tiles/home.jsp">Home</a></li> <li><a href="/tiles/about.jsp">About us</a></li> <li>Menu item 3</li> <li>Menu item 4</li> <li>Menu item 5</li> <li>Menu item 6</li> </ul> </div>
For our second page, I only have to code the body, reusing the other tiles (header, footer, and menu). Here is how the about page looks like :
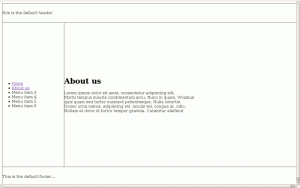
The about page
Tiles inheritance
Now, looking at the configuration file, and predicting my application will grow with lots of pages using the exact same template, I see annoying redundancy in defining the pages. As an example, if all my pages use the same header, I don’t want to specify it each time I define a new page. More than that, why should I write template=”template.jsp” on each tiles definition, when I know I always use the same template?
Tiles allows the developper to inherit (extend) an abstract definition :
<?xml version="1.0" encoding="ISO-8859-1" ?> <!DOCTYPE tiles-definitions PUBLIC "-//Apache Software Foundation//DTD Tiles Configuration 2.0//EN" "http://tiles.apache.org/dtds/tiles-config_2_0.dtd"> <tiles-definitions> <definition name="commonPage" template="/template.jsp"> <put-attribute name="header" value="/defaultHeader.jsp" /> <put-attribute name="menu" value="/defaultMenu.jsp" /> <put-attribute name="footer" value="/defaultFooter.jsp" /> </definition> <definition name="homePage" extends="commonPage"> <put-attribute name="body" value="/home_body.jsp" /> </definition> <definition name="aboutPage" extends="commonPage"> <put-attribute name="body" value="/about_body.jsp" /> </definition> </tiles-definitions>
Tiles configuration can be manipulated in many other ways, such as using subdefinitions or nested definitions (see Apache Tiles, Nested definitions).
Next time I look upon Tiles, I probably will study how it integrates with the Spring MVC framework.
Many many Thanks!
It was the best Tiles tutorial what I’ve found!
Congratulations!
A.
Comment by Alice — March 14, 2009 @ 12:50 am |
Thank you Alice, you’re too kind :)
Comment by baraber — March 14, 2009 @ 1:15 am |
It’s really so, I’ve “googled” 10 hours to find the adequate tutorial to strat with tiles!
But I’ve now a silly problem. (Perhaps because I haven’t enough time to do all of your examples)
I tried to bootstrap your Springs an Tiles application, but I couldn’t because I’ve a mapping error(I suppose) I’ve the exeption :
SEVERE: StandardWrapper.Throwable
org.springframework.beans.factory.BeanCreationException: Error creating bean with name ’tilesConfigurer’ defined in ServletContext resource [/WEB-INF/applicationContext.xml]: Invocation of init method failed; nested exception is org.apache.tiles.definition.DefinitionsFactoryException: Unable to parse definitions from /WEB-INF/defs/myTilesConfigFile.xml
at org.springframework.beans.factory.support.AbstractAutowireCapableBeanFactory.initializeBean
…
Could you send me your directory structure?
Thank you in anticipation
Alice
Comment by Alice — March 15, 2009 @ 2:33 pm |
Hello Alice.
I played around with my project and have been able to reproduce your problem in the case that myTilesConfigFile.xml is not found. If this definition file is really under yourProjetDirectory/WEB-INF/defs, than your tilesConfigurer definition should be as follow :
<bean id=”tilesConfigurer” class=”org.springframework.web.servlet.view.tiles2.TilesConfigurer”>
<property name=”definitions”>
<list>
<value>/WEB-INF/defs/myTilesConfigFile.xml</value>
</list>
</property>
</bean>
If this doesn’t help, maybe you could post your applicationContext.xml content or anymore details that could help me help you. But it would be better if you do so in the comments thread of the Spring-Tiles integration post. Since there, wish you good luck :)
Comment by baraber — March 15, 2009 @ 6:35 pm |
Hello Richard!
Thank you very much for the rash answer, I go over with my reaction to the other theard
Alice
Comment by Alice — March 15, 2009 @ 7:56 pm |
Hi Richard,
This is a simple and nice tutorial. Thank you for sharing.
Comment by Manjula — March 24, 2009 @ 5:26 am |
Hi Richard,
I too am trying to integrate Spring 2.5.6 with Tiles 2.1.2 and was very grateful to find your solution. I’ve copied your SpringTileConfigurer class but when I’m getting an exception thrown as follows. I can’t find much information about this and was wondering if you had maybe seen it yourself and may be able to help. Am I using the corret viewResolver ?
<bean id=”viewResolver” class=”org.springframework.web.servlet.view.UrlBasedViewResolver”<
<property name=”viewClass” value=”org.springframework.web.servlet.view.tiles2.TilesView”/<
</bean>
<bean id=”tilesConfigurer” class=”com.freebee.utils.SpringTilesConfigurer”>
<property name=”definitions”>
<list>
<value>WEB-INF/freebee-tiles.xml</value>
</list>
</property>
</bean>
many thanks
org.springframework.web.util.NestedServletException: Request processing failed; nested exception is javax.el.ELException: Expression cannot be null
org.springframework.web.servlet.FrameworkServlet.processRequest(FrameworkServlet.java:583)
org.springframework.web.servlet.FrameworkServlet.doGet(FrameworkServlet.java:501)
javax.servlet.http.HttpServlet.service(HttpServlet.java:617)
javax.servlet.http.HttpServlet.service(HttpServlet.java:717)
org.jboss.web.tomcat.filters.ReplyHeaderFilter.doFilter(ReplyHeaderFilter.java:96)
root cause
javax.el.ELException: Expression cannot be null
org.apache.el.lang.ExpressionBuilder.createNodeInternal(ExpressionBuilder.java:87)
org.apache.el.lang.ExpressionBuilder.build(ExpressionBuilder.java:150)
org.apache.el.lang.ExpressionBuilder.createValueExpression(ExpressionBuilder.java:194)
org.apache.el.ExpressionFactoryImpl.createValueExpression(ExpressionFactoryImpl.java:68)
org.apache.tiles.evaluator.el.ELAttributeEvaluator.evaluate(ELAttributeEvaluator.java:156)
org.apache.tiles.evaluator.AbstractAttributeEvaluator.evaluate(AbstractAttributeEvaluator.java:44)
org.apache.tiles.renderer.impl.AbstractBaseAttributeRenderer.render(AbstractBaseAttributeRenderer.java:101)
org.apache.tiles.impl.BasicTilesContainer.render(BasicTilesContainer.java:659)
org.apache.tiles.impl.BasicTilesContainer.render(BasicTilesContainer.java:678)
org.apache.tiles.impl.BasicTilesContainer.render(BasicTilesContainer.java:633)
org.apache.tiles.impl.BasicTilesContainer.render(BasicTilesContainer.java:322)
org.springframework.web.servlet.view.tiles2.TilesView.renderMergedOutputModel(TilesView.java:75)
org.springframework.web.servlet.view.AbstractView.render(AbstractView.java:257)
org.springframework.web.servlet.DispatcherServlet.render(DispatcherServlet.java:1183)
org.springframework.web.servlet.DispatcherServlet.doDispatch(DispatcherServlet.java:902)
org.springframework.web.servlet.DispatcherServlet.doService(DispatcherServlet.java:807)
org.springframework.web.servlet.FrameworkServlet.processRequest(FrameworkServlet.java:571)
org.springframework.web.servlet.FrameworkServlet.doGet(FrameworkServlet.java:501)
javax.servlet.http.HttpServlet.service(HttpServlet.java:617)
javax.servlet.http.HttpServlet.service(HttpServlet.java:717)
org.jboss.web.tomcat.filters.ReplyHeaderFilter.doFilter(ReplyHeaderFilter.java:96
Comment by Paul — March 25, 2009 @ 4:23 pm |
Thanks so much, this really is the best Tiles tutorial on the whole web, bar none! Apache’s tutorial is full of mistakes, is impractical, and vague. I spent about 3 days trying to learn Tiles and fix things following Apache’s tutorial. Took about 5 minutes (no exaturation!) to get it with your tutorial!!!
Comment by Hamman — April 7, 2009 @ 9:38 am |
Yeah; its the same case i passed a lot of days in this subjet; but with your tutorial it was faster ;) thanks very much
Comment by Francisco — April 14, 2009 @ 11:53 am |
Hello!
I have been working with struts2 for a while and stated I would like to start developing with Tiles2.
Because it would help me to get rid of allot of copy and paste of code. Unfortunately Tile2 were not that easy to understand as I thought before I started. I see here that you got a pretty detailed implementation, and I tried it out for my self. But it didn’t work for me, don’t really know were I went wrong.
Wonder if you could send me your sample code, thanks in regards!
Comment by Ale — April 28, 2009 @ 11:05 am |
Hey, nice tutorial dude…
Comment by Avi — May 6, 2009 @ 8:44 am |
Richard,
This is a very nice example. I’ve used an older version of tiles with struts and could not find a nicer example than yours explaining strust 2.0 way.
great job.
Comment by Raad — May 22, 2009 @ 12:11 pm |
Great Job, this is really the best work for Tiles.
Here is my question, how do we eliminate using 2 JSP for one
result. In this example, we use home.jsp and home_body jsp.
Therefore, if we have 100 pages , we will end up creating
200 pages…Or Am I missing something here?
Again, Good work…
Comment by Ed — July 1, 2009 @ 3:04 pm |
Your tutorial is great. Apaches own tutorial on tiles SUCKS
Comment by Frederick — July 25, 2009 @ 1:02 am |
Thank you, this is really very helpful. well explained.
Comment by Ragasudha — August 13, 2009 @ 3:33 am |
[…] […]
Pingback by Tiles Template + JSF-Navigation - Enterprise Java (JEE, J2EE, Spring & Co.) @ tutorials.de: Forum, Tutorial, Anleitung, Schulung & Hilfe — August 18, 2009 @ 10:47 am |
Great tutorial, maybe the best in the network… sure…. Thank you….
Comment by nicola — September 4, 2009 @ 11:07 am |
That was so helpful Thank you !
Comment by jrp — December 4, 2009 @ 3:56 am |
A sigh of relief ……i started hated tiles when i was not getting it work as provided by apache tiles tutorials but your tutorial comes as a booster…..now nobody stop me making my own site in java ee
Comment by Anup — March 1, 2010 @ 1:36 am |
Perfect explanation of the concept! Thank you very much!
Comment by Eugene — March 10, 2010 @ 1:49 pm |
Hi,
I like this tutorial, thank you so much, we appreciate your helps,
do you have a war file or the source code of this example.
thanks
Comment by Majid — March 30, 2010 @ 11:48 am |
Thanks for this tutorial. This tutorial is very easy and simple to understand.
Comment by duy khoa — April 24, 2010 @ 2:11 pm |
Taaa very much body!!!, like Alice and the others, i spent a lot of time looking for the good tutorial even if the official documentation is available and clear(not very clear…), i tried the configuration tiles with servlet in the “web.xml” but it didn’t work(TilesServlet and “…TilesContainer not initialized” problems).
anyway, i’m so greatful and thank you again ;)
Comment by pureself — May 4, 2010 @ 12:32 pm |
Nicely done, Richard. The tutorial on the Apache site is stilted and horrible. Your tutorial has great economy and simplicity. Thanks.
Comment by Clark — May 4, 2010 @ 4:07 pm |
The matchless phrase, is pleasant to me :)
Comment by extract audio from video — July 10, 2010 @ 11:18 am |
Hi,
i have tried everything as you mentioned but i am getting 404 error after running the application on eclipse .. please can you tell me the possible mistakes..
Comment by felcon — September 2, 2010 @ 1:41 am |
Great buddy…
As some one mentioned above the example given on apache site is very difficult to understand..
You made it very clear..
Thanks for teaching me how tiles works ;)
Comment by Ramesh — September 17, 2010 @ 11:52 pm |
Thanks for sharing Richard, this is the best tutorial about tiles I’ve found after spending hours on the web !!! It is really amazing !
Comment by Jack — October 6, 2010 @ 5:13 am |
A million thanks to you for explaining Tiles in such a lucid way.
Comment by Nilesh — November 1, 2010 @ 2:12 am |
Great tutorial ! Helped me lot in jumpstarting my project ! Keep up the good work !
Comment by ganesh — November 1, 2010 @ 5:52 am |
Nice Tutorial
Comment by Naveen Reddy — November 10, 2010 @ 6:15 pm |
Hi Richard,
As a beginner for tiles, with your tutorial I got it up and running with in minutes. Thanks a lot for that. And as a maven user i had to include following dependencies to my pom.xml file
org.slf4j
slf4j-api
1.5.5
org.apache.tiles
tiles-extras
2.2.2
Comment by sameera — November 25, 2010 @ 1:46 am |
Thanks Good Tutorial and Thanks for the Maven tips
Keep up the good work
Comment by Farshid — November 11, 2011 @ 12:11 am |
Hi Richard,
Just wanted to let you know that Spring 3.0.5 with Tiles 2.2 and it seems to work without any patch in TilesConfigurer. Thanks for posting this article.
Sam
Comment by Samuel Contesse — January 19, 2011 @ 4:16 pm |
This is very helpful post
Comment by Sankar R K — February 7, 2011 @ 3:02 am |
Hi,
I want to know what should I return at my Controllers to make use of tile based views instead of JSP based views (which are the project default).
Thanks
Comment by Luís Soares — July 20, 2011 @ 11:43 am |
I would really like to see a Portlet equivalent.
Comment by Mike Oliver — September 14, 2011 @ 8:59 pm |
Hi Richard,
Nice tutorial for beginner.
Comment by Pawan — September 21, 2011 @ 2:17 am |
Thanks for your clear, easy and yet sufficient introduction to the subject!
Having to take over maintenance of an old frames based application your introduction was the perfect companion.
Anders
Comment by Anders — October 29, 2011 @ 4:14 am |
Thanks for the wonderful tutorial. I have tried the same code but I could not successfully open the home.jsp.
I am getting the following error. Any idea about the error.
BasicTilesCon W org.apache.tiles.impl.BasicTilesContainer render Unable to find the definition ‘homePage’
[1/24/12 13:45:15:198 EST] 00000012 RoleSecurityT E org.apache.tiles.jsp.taglib.RoleSecurityTagSupport doEndTag Error executing tag: homePage
org.apache.tiles.definition.NoSuchDefinitionException: homePage
at org.apache.tiles.impl.BasicTilesContainer.render(BasicTilesContainer.java:578)
at org.apache.tiles.impl.BasicTilesContainer.render(BasicTilesContainer.java:246)
at org.apache.tiles.jsp.taglib.InsertDefinitionTag.render(InsertDefinitionTag.java:63)
at org.apache.tiles.jsp.taglib.RenderTagSupport.execute(RenderTagSupport.java:171)
at org.apache.tiles.jsp.taglib.RoleSecurityTagSupport.doEndTag(RoleSecurityTagSupport.java:75)
thanks
Comment by shaki — January 24, 2012 @ 2:47 pm |
Hi Richard,
Thank you so much for writing this article. I gave off my entire evening yesterday to solve this problem and was unable to find the solution for it. Its 7 AM in the morning, and I saw your article, you saved my day.
Thank You.
Comment by Akshay Sahu — February 1, 2012 @ 11:13 pm |
one noob question. In the last example that you have provided for extending an existing tile, how do we specify where the body tile is supposed to appear in the template. There are three other tiles there (head,menu,footer) so where will the body tile appear?
Comment by Amit Rai — February 3, 2012 @ 10:14 am |
tried many tiles use samples – None of them working properly,.. missing methods, version incompatibilities, initialization fail,…
Here is alternative simple and standard use of tags to reproduce the same “Tiled View” but with no tiles libraries and special XML configurations
<!—->
<!—->
<!–
OR you may dynamically assign include page url in session bean within MVC process
..
// portalBean.setPageUrl(“home_body.jsp”) ;
..
<jsp:include page="”>
<!– –>
in addition:
any server-side logic (servlets, controllers) presented within jsp page will be processed before the include loaded into the parent frame.
What means for instance in the case of menu, that it can be in turn dynamically processed by some menu controller and than loaded into frame placeholder
in general
works with JSP, ASP (ASP.NET), PHP…
Comment by byrop — February 14, 2012 @ 11:09 am |
Thanks…Was great help…
Comment by Hiren — June 28, 2012 @ 7:07 am |
Thanks a Lot…..!
It was the best Tiles tutorial what I’ve found!
Comment by Manish Kumar — August 8, 2012 @ 1:33 pm |
Valuable info. Lucky me I discovered your web site unintentionally, and
I’m surprised why this accident did not took place earlier! I bookmarked it.
Comment by how to post vimeo videos in wordpress, — September 15, 2012 @ 6:22 am |
good example.its very helpful for me
Comment by Aswathi — October 14, 2012 @ 2:45 am |
Many thanks for the tutorial, which is so missing in tiles doc.
I used Tiles with Struts about 6 years ago with ease and satisfaction. Now I want to use Tiles 3 in standalone with Servlets, supposed to be the simplest way to use it. But no way to make in work after solving countless NoClassDefFound due to all jars dependencies ( comparing with sitemesh unique jar !). Very desapointed with the documentation. After half a day trying to understand the documentation and make it work. Blank page no error in logs ! I step away. This project should be removed from Apache. Its disapointing and enlighting: After years of development, projects should improve, but Tiles is getting worse and worse.
Comment by Thierry — October 27, 2012 @ 1:29 pm |
Awesome….
Comment by Sidhartha — May 7, 2013 @ 10:35 pm |
[…] I am trying to run sample tiles example given here […]
Pingback by NoClassDefFoundError: org/slf4j/impl/StaticLoggerBinder | Ask Programming & Technology — November 4, 2013 @ 2:00 am |
Thanks for your lucid explanation!
Comment by Solomon — February 10, 2014 @ 2:53 pm |
Hai Ur tutorial helped me a lot for learning basic tiles framework example without using any other frameworks.Thanks a lot for you !!!:)
Comment by Manikandan Arunachalakani — September 19, 2014 @ 6:55 am |
The way you came through is very simple and quite easy to understand
Thanks a lot….
Here is an example war which we have tried.
https://drive.google.com/file/d/0B8fvYihmCI2oR2o0SlF1b1pwbEU/edit?usp=sharing
Comment by Sasi Kumar — September 19, 2014 @ 7:06 am |